Textarea
テキストなどの情報を複数行入力するためのコンポーネントです。textarea
の代わりとして使用します。入力文字数を数える機能や入力によって自動で領域が広がる機能を備えています。
使用上の注意
適切なサイズに調整して使用する
入力する内容をあらかじめ精査し適切なサイズになるよう調整して使用してください。
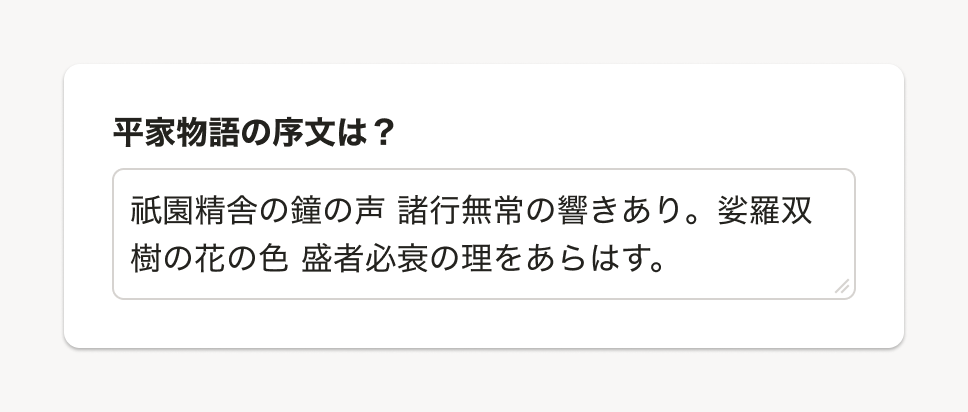
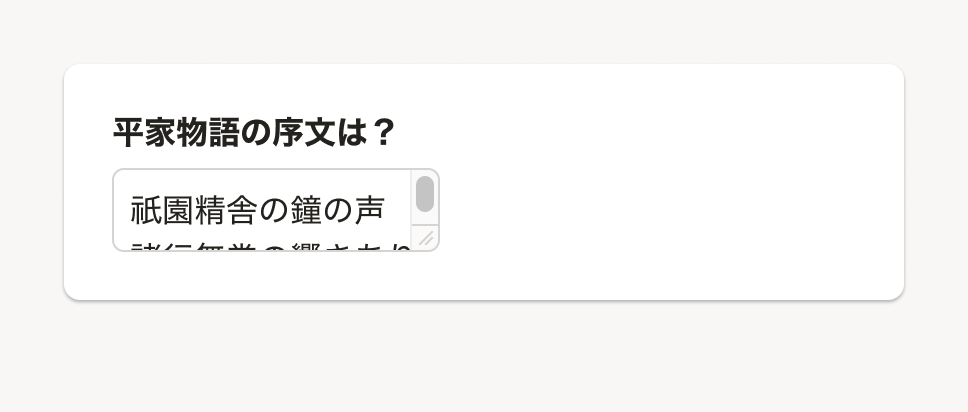
適切なサイズは以下の判断基準を参考にしてください。
- Textareaはデフォルトで2行分の高さが確保され、幅を超えると改行されますが、幅と高さが小さいと入力内容が確認しづらくなります。十分なサイズを確保してください。幅は
witdh
props、高さはrows
propsで指定します。 - 入力に依って自動で高さが広がって良い場合は、
autoResize
propsを指定します。 - 高さを自動て広げつつ上限を設定する場合は、
maxRows
propsを指定します。 - 入力する内容の文字数の予測が付く場合、過剰に高さを確保しないでください。わかりづらくなったり、視線誘導の妨げになってしまうことがあります。
- フォーム全体で見たときにレイアウトに統一性がなく感じられるときは、他の要素とのバランスを考慮してサイズを調整してください。
入力できる文字数に上限がある場合は入力文字数を表示する
入力できる文字数の上限が決まっている場合はmaxLetters
propsを指定し、上限に応じた幅と高さに調整してください。
ユースケースに応じてコンポーネントを使い分ける
一行のテキスト入力が想定される場合は使用しない
氏名やメールアドレスなどの一行のテキスト入力にはInputを使用してください。
データの編集や送信を伴わない画面では使用しない
データの編集や送信を伴わない画面でデータを表示する場合はDefinitionListを使用してください。
入力項目の説明や例をプレースホルダで表示しない
入力項目の説明や例を載せる場合はFormControlのヘルプメッセージ(helpMessage
)や入力例(exampleMessage
)などを用い別途表示してください。
状態
無効(disabled)
入力を受け付けない状態を表現したスタイルです。通常は編集ができるが一時的または権限の制約により編集ができない場合に使用します。
ユーザーはなぜ無効になっているのかわからないことがあります。権限による表示制御のデザインパターンを参考にして、そもそも無効ではなくフォーム自体を非表示にしたり、無効状態の理由を付近に表示することを検討してください。
Props
自動でフォーカスされるかどうか
@deprecated placeholder属性は非推奨です。別途ヒント用要素の設置を検討して ください。
行数の初期値。省略した場合は2
入力値にエラーがあるかどうか
コンポーネントの幅
自動で広がるかどう か
最大行数。超えるとスクロールする。初期値は無限
入力可能な最大文字数。あと何文字入力できるかの表示が追加される。html的なvalidateは発生しない
コンポーネント内の文言を変更するための関数を設定